all about vlsi DV
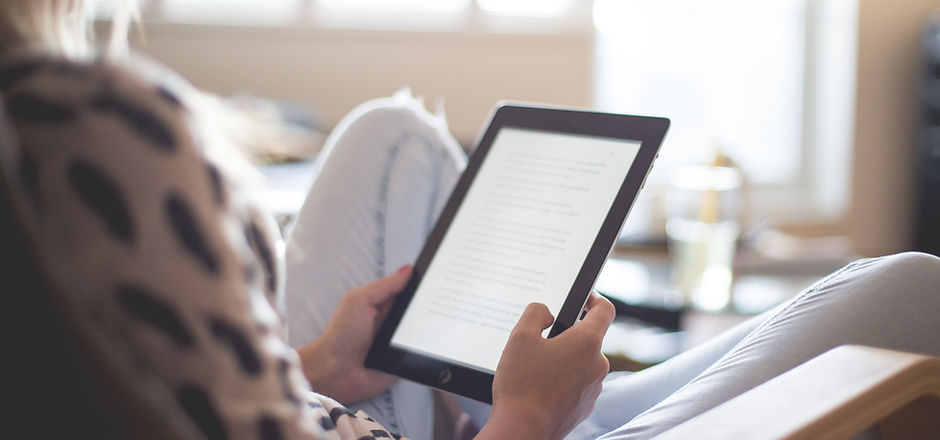
First assembly language program
​
To get started without any tool hiccups we will be using online tool. Open : https://cpulator.01xz.net/?sys=arm
Let's go through the provided minimal ARM assembly program line by line:
```assembly
.global _start // Declare the start of the program and make _start visible to the linker
```
- This line is a directive indicating that `_start` is a global symbol and should be visible to the linker. The linker uses this information to know where the program execution should begin.
```assembly
.section .text // Start of the code segment
_start: // Program entry point
```
- The `.text` directive indicates the beginning of the code segment, where the actual instructions of the program are placed.
- `_start` is a label representing the entry point of the program. The program execution begins here.
```assembly
// Halt the program
mov r7, #1 // Move the value 1 into register r7
swi 0 // Trigger a software interrupt to terminate the program
```
- `mov r7, #1`: This instruction moves the immediate value 1 into register `r7`. In this case, it is preparing the value that will be used to specify the type of action in the software interrupt.
- `swi 0`: This instruction triggers a software interrupt (SWI) with a software interrupt number of 0. SWI 0 is commonly used as a convention to request an operating system service or to terminate a program gracefully. In this minimal program, it serves as a way to halt the execution.
​
A bit about types of Registers in ARM Architecture:
ARM architecture has several types of registers, categorized based on their usage:
1. **General-Purpose Registers (R0-R15):**
- R0 to R12: General-purpose registers used for various purposes.
- R13 (SP): Stack Pointer, used to manage the program stack.
- R14 (LR): Link Register, used to store the return address when calling subroutines.
- R15 (PC): Program Counter, holds the address of the next instruction to be executed.
2. **Status Registers:**
- CPSR (Current Program Status Register): Contains flags and other status information.
3. **Banked Registers:**
- Some registers have banked versions that are used in different processor modes (e.g., IRQ, Supervisor, etc.).
4. **Floating-Point Registers:**
- F0 to F31: Floating-point registers used for floating-point operations.
5. **Vector Registers:**
- Q0 to Q31: Quadword registers for Advanced SIMD (NEON) operations.
In the provided minimal program, we use a general-purpose register (`r7`) to hold the value 1, and the program utilizes the Program Counter (`r15`) implicitly as it executes the SWI instruction. The specifics can vary based on the ARM architecture version and the context of the program.
​
​
Simple tasks:
Can you modify the program to update all the register values?
Also play around by executing each instruction one after another and note the changes in PC values.